These layers are described below.
1. Application layer or Business layer
2. Business layer
a. Property layer(Sub layer of business layer)
3. data layer
Advantages of three Tier Architecture.
The main characteristic of a Host Architecture is that the application and databases reside on the same host computer and the user interacts with the host using an unfriendly and dump terminal. This architecture does not support distributed computing (the host applications are not able to connect a database of a strategically allied partner). Some managers found that developing a host application take too long and it is expensive. Consequently led these disadvantages to Client-Server architecture.
Client-Server architecture is 2-Tier architecture because the client does not distinguish between Presentation layer and business layer. The increasing demands on GUI controls caused difficulty to manage the mixture of source code from GUI and Business Logic (Spaghetti Code). Further, Client Server Architecture does not support enough the Change Management. Let suppose that the government increases the Entertainment tax rate from 4% to 8 %, then in the Client-Server case, we have to send an update to each clients and they must update synchronously on a specific time otherwise we may store invalid or wrong information. The Client-Server Architecture is also a burden to network traffic and resources. Let us assume that about five hundred clients are working on a data server then we will have five hundred ODBC connections and several ruffian record sets, which must be transported from the server to the clients (because the Business layer is stayed in the client side). The fact that Client-Server does not have any caching facilities like in ASP.NET, caused additional traffic in the network. Normally, a server has a better hardware than client therefore it is able compute algorithms faster than a client, so this fact is also an additional pro argument for the 3.Tier Architecture. This categorization of the application makes the function more reusable easily and it becomes too easy to find the functions which have been written previously. If programmer wants to make further update in the application then he easily can understand the previous written code and can update easily.
Application layer or Presentation layer
Application layer is the form which provides the user interface to either programmer of end user. Programmer uses this layer for designing purpose and to get or set the data back and forth.
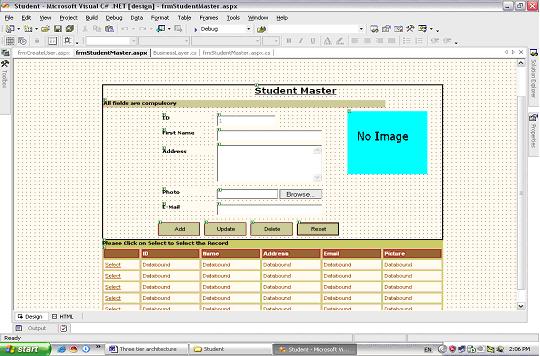
Business layer
This layer is a class which we use to write the function which works as a mediator to transfer the data from Application or presentation layer data layer. In the three tier architecture we never let the data access layer to interact with the presentation layer.
a. Property Layer
This layer is also a class where we declare the variable corresponding to the fields of the database which can be required for the application and make the properties so that we can get or set the data using these properties into the variables. These properties are public so that we can access its values.
Data Access Layer
This layer is also a class which we use to get or set the data to the database back and forth. This layer only interacts with the database. We write the database queries or use stored procedures to access the data from the database or to perform any operation to the database.Summary
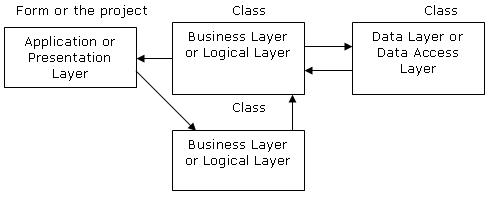
Application layer is the form where we design using the controls like textbox, labels, command buttons etc.
Business layer is the class where we write the functions which get the data from the application layer and passes through the data access layer.
Data layer is also the class which gets the data from the business layer and sends it to the database or gets the data from the database and sends it to the business layer.
Property layer is the sub layer of the business layer in which we make the properties to sent or get the values from the application layer. These properties help to sustain the value in a object so that we can get these values till the object destroy.
Data flow from application layer to data layer
You can download sample three tier project, used for this tutorial. Here we are passing the code of the student to the business layer and on the behalf of that getting the data from the database which is being displayed on the application layer.
Presentation Layer:
private void DataGrid1_SelectedIndexChanged(object sender, System.EventArgs e)
{
// Object of the Property layer
clsStudent objproperty=new clsStudent();
// Object of the business layer
clsStudentInfo objbs=new clsStudentInfo();
// Object of the dataset in which we receive the data sent by the business layer
DataSet ds=new DataSet();
// here we are placing the value in the property “ID†using the object of the
property layer
objproperty.id=int.Parse(DataGrid1.SelectedItem.Cells[1].Text.ToString());
// In ths following code we are calling a function from the business layer and passing the object of the property layer which will carry the ID till the
database.
ds=objbs.GetAllStudentBsIDWise(objproperty);
// What ever the data has been returned by the above function into the dataset is
being populate through the presentation laye.
txtId.Text=ds.Tables[0].Rows[0][0].ToString();
txtFname.Text=ds.Tables[0].Rows[0][1].ToString();
txtAddress.Text=ds.Tables[0].Rows[0][2].ToString();
txtemail.Text=ds.Tables[0].Rows[0][3].ToString();
Image1.ImageUrl=ds.Tables[0].Rows[0][4].ToString();
}
Property Layer
// These are the properties has been defined in the property layer. Using the object of the property layer we can set or get the data to or from these properties.
public class clsStudent // Class for Student Table
{
private int _id;
private string _Name;
private string _Address;
private string _Email;
private string _Picture;
public int id // Property to set or get the value into _id variable
{
get{return _id;}
set{_id=value;}
}
public string Name
{
get{return _Name;}
set{_Name=value;}
}
public string Address
{
get{return _Address;}
set{_Address=value;}
}
public string Email
{
get{return _Email;}
set{_Email=value;}
}
public string Picture
{
get{return _Picture;}
set{ _Picture=value;}
}
}
Business Layer:
"Obj" is the object of the clsStudent class has been defined in the property layer. This function is receiving the property object and passing it to the datalayer class
// this is the function of the business layer which accepts the data from the application layer and passes it to the data layer.
public class clsStudentInfo
{
public DataSet GetAllStudentBsIDWise(clsStudent obj)
{
DataSet ds=new DataSet();
ds=objdt.getdata_dtIDWise(obj);// Calling of Data layer function
return ds;
}
}
Datalayer Layer
// this is the datalayer function which is receiving the data from the business layer and
performing the required operation into the database
public class clsStudentData // Data layer class
{
public DataSet getdata_dtIDWise(clsStudent obj) // object of property layer class
{
DataSet ds;
string sql;
sql="select * from student where StudentId="+obj.id+" order by StudentId";
ds=new DataSet();
// this is the datalayer function which accepts trhe sql query and performs the
corresponding operation
ds=objdt.ExecuteSql(sql);
return ds;
}
}
For More Information:- http://www.beansoftware.com/ASP.NET-Tutorials/Three-Tier-Architecture.aspx